How to add a live avatar stack to your product with React, Firebase, and Liveblocks
Learn how you can create and add a live avatar stack to your product using React, Firebase, and Liveblocks.
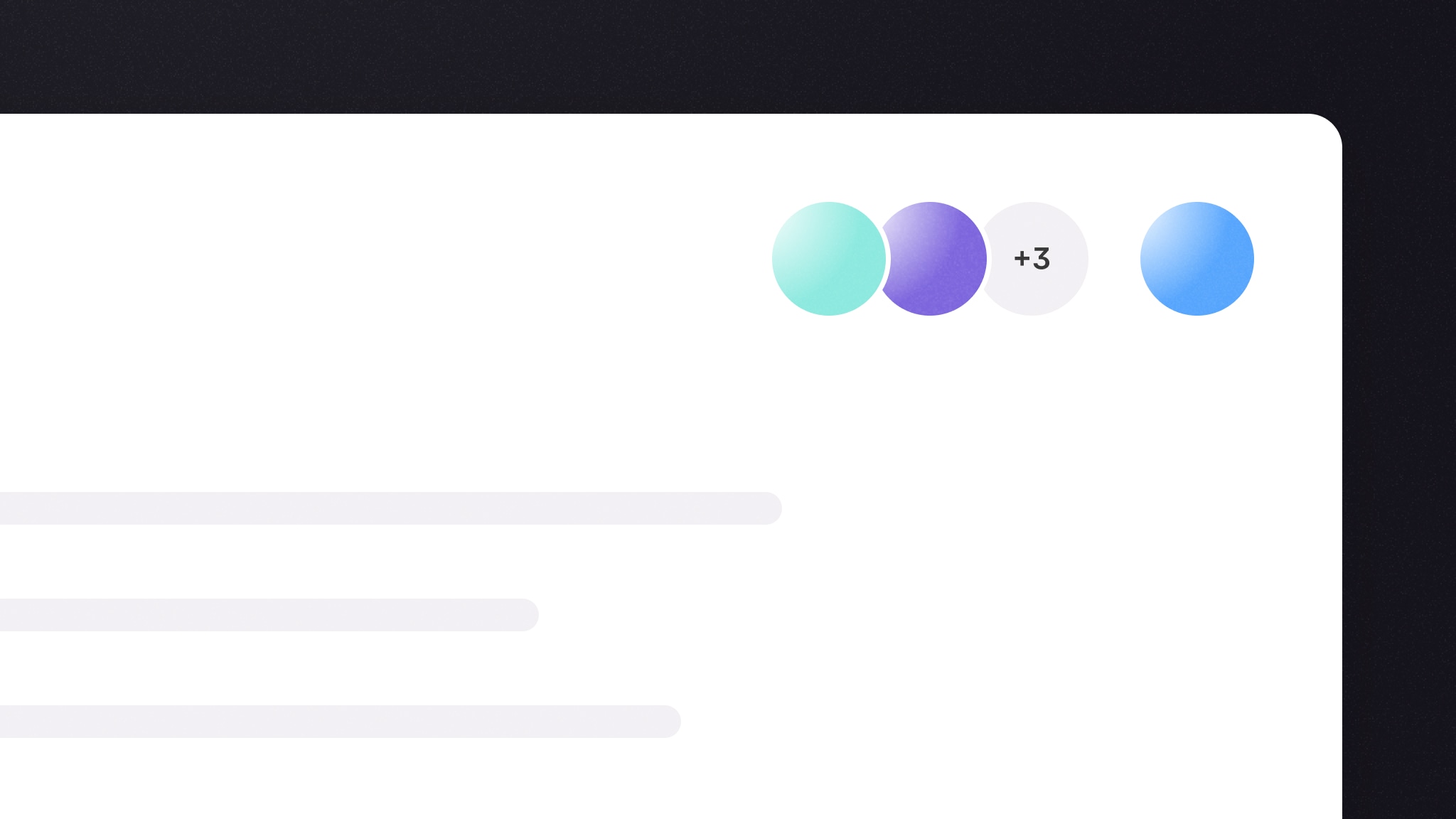
Aim
This tutorial aims to guide you on how you can create and add a live avatar stack to your product using React, Firebase, and Liveblocks. By the end of this tutorial, you should have a solid understanding of:
- What a live avatar stack is
- What Liveblocks is
- How to install and connect to Liveblocks
- How to use Firebase in Liveblocks for authentication
- A few Liveblocks React hooks such as
useOthers()
anduseSelf()
Prerequisites
In this guide, we assume that you already have a decent understanding of:
- React and React Hooks,
- Firebase and Firebase functions,
- Javascript Async functions and Higher order functions such as array
map()
function. - Basic Node.js and npm for you to follow along easily.
Also, you need to create a Liveblocks account, sign in to your dashboard and retrieve your API key (secret key) which will be used in this tutorial.
What a live avatar stack is
A live avatar is a pictorial representation of a collaborator working in a virtual space. Applications like Figma and Dropbox use live avatars to represent active users. When the user enters the virtual space, an avatar is created to indicate the user’s presence, and also when the user leaves, the avatar is removed or dimmed to indicate the user’s absence. This helps collaborators to work as though they were in a physical room and can see who is present or not. An avatar stack is a group of avatars and here’s an image of what a typical avatar stack looks like:
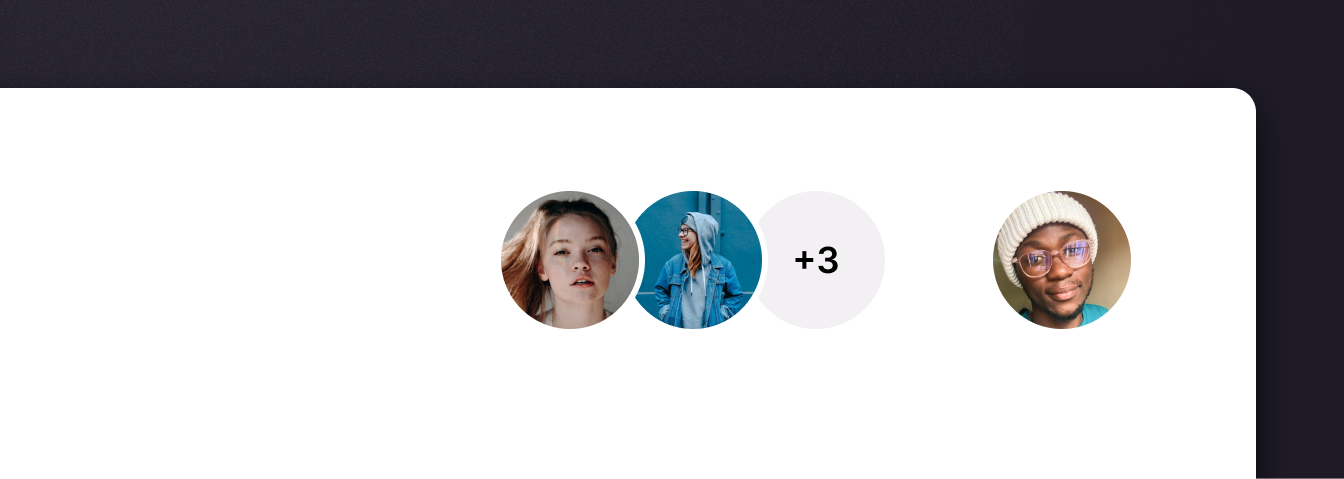
Add Liveblocks to your project
To add Liveblocks to your React project, you first need to install Liveblocks packages into your project. To do that please run the following command:
@liveblocks/client
lets you connect
to Liveblocks servers.
@liveblocks/react
contains react
utilities to make it easier to consume @liveblocks/client
.
Connect to Liveblocks server
Next, you need to connect to Liveblocks server using the LiveblocksProvider
component in the root of your project (let’s say index.js
file) and pass in
client
as a prop to this component. Remember this makes Liveblocks hooks
available to all of your components wrapped in the LiveblocksProvider
component. This is a typical example of what your root file (say index.js
file) should look like:
Connect to Liveblocks room
Remember a room (the virtual space) where users collaborate? The next step is to
connect to this room and to do this, we use the RoomProvider
in our App.js
component and set a "live-avatars" ID like so:
Set-up authentication endpoint using Firebase
Remember you can make use of your Liveblocks public key or an authentication
endpoint to create a client using the createClient
function. We will be
creating an authEndpoint using Firebase. We assume that you have set up your
Firebase config and added Firebase to your project. Users can only interact with
rooms they have access to. You can control permission access from an http
endpoint by creating a
Firebase function. To
do that, let’s first install @liveblocks/node
package in your Firebase
functions project.
Next, create a new Firebase
callable function (let’s
say auth
) as described below. This is where you have to implement your
security and define if the current user has access to a specific room. Don’t
forget to replace sk_prod_xxxxxxxxxxxxxxxxxxxx
with
your API key.
We’ve successfully set up our Firebase authentication endpoint!
Create a live avatar stack UI
Here’s the representation of what our avatar stack structure would look like:
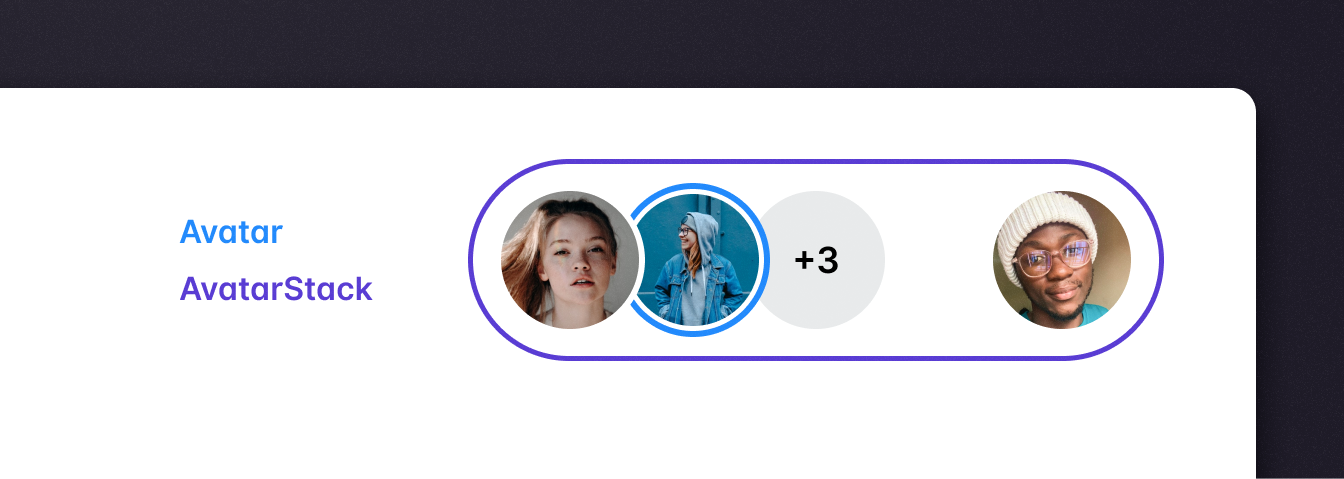
Avatar component
This is what our Avatar.js
component should look like:
And here is the Avatar.module.css
file to go alongside with it:
AvatarStack component
This is what our AvatarStack.js
component should look like:
And here is the AvatarStack.module.css
file to go alongside with it:
Conclusion
And there you have it! Your AvatarStack
component is available to be imported
and used however and wherever you wish to use it in your product.
Liveblocks makes it easy to implement online/virtual presence to improve collaboration between team members. However, a live avatar stack isn’t the only presence UI pattern you can build with Liveblocks, you can also build things like live cursors, live selection, and more. Thank you for your time!
Ready to get started?
Join thousands of companies using Liveblocks ready‑made collaborative features to drive growth in their products.