How to add users to Liveblocks Comments
After following the get started guide for Comments, you’ll notice that each user is currently “Anonymous”, and that there’s no way to mention or tag other users. To enable these features, we need to tell Comments where to find your users’ information.
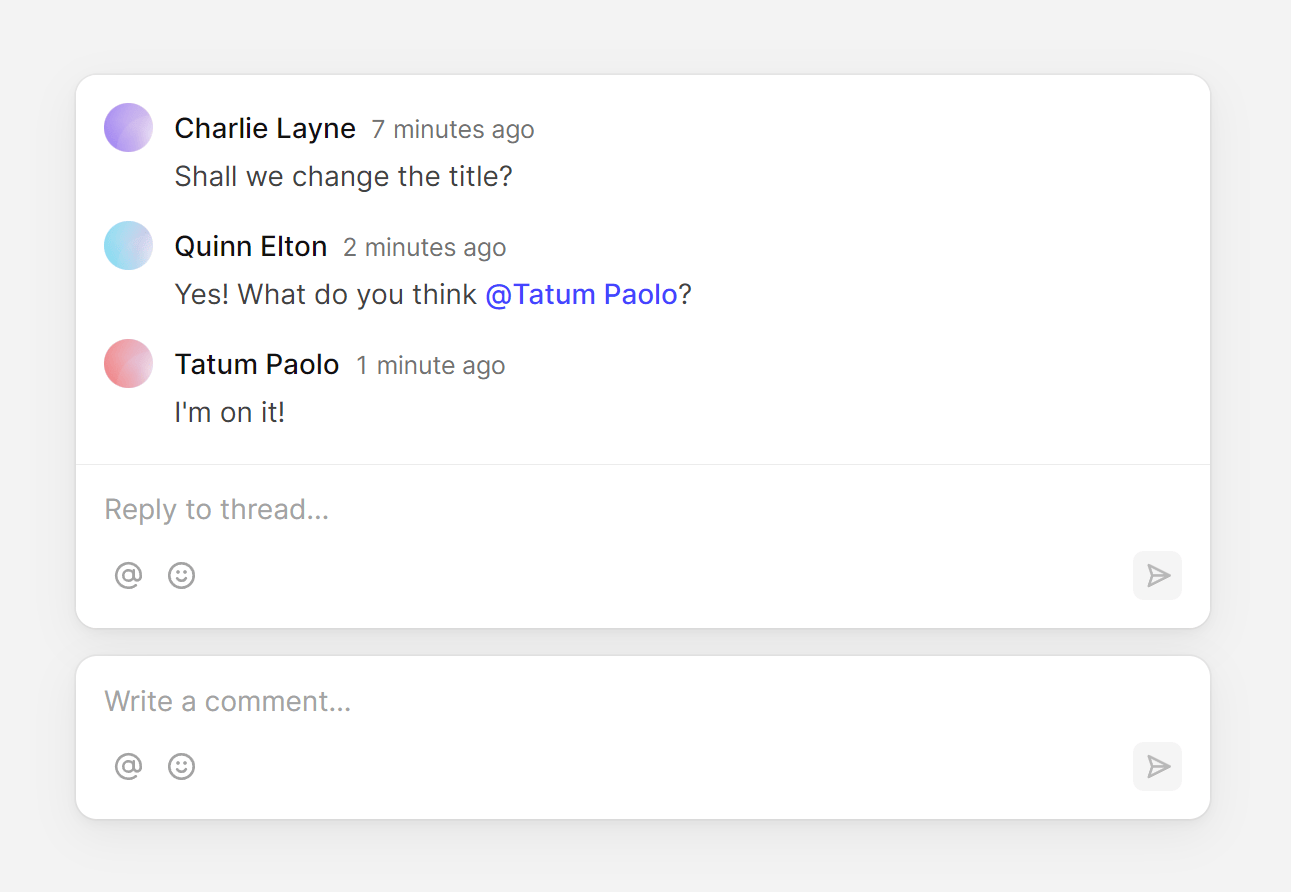
What we’re learning
In this guide we’ll be modifying
LiveblocksProvider
,
learning how to:
- Add names and avatars to threads and comments with
resolveUsers
. - Create user mention suggestions in the composer using
resolveMentionSuggestions
.
Authenticate your application
The first step is to find an authentication guide for your framework and authenticate your app, as this is necessary for Comments.
Make sure to follow the metadata step in the guide, and attach the name of your user, along with the URL of their avatar, as these properties will both be used in the default components. Here’s an example using access token authentication, with an email address as a user’s ID.
If you’re using ID token authentication, it’ll look a little different.
Don’t forget to modify your UserMeta
type in liveblocks.config.ts
to match
the metadata format, adding type hints to your editor.
Resolving users
To show each user’s name and avatar in threads and comments, we need to use
resolveUsers
.
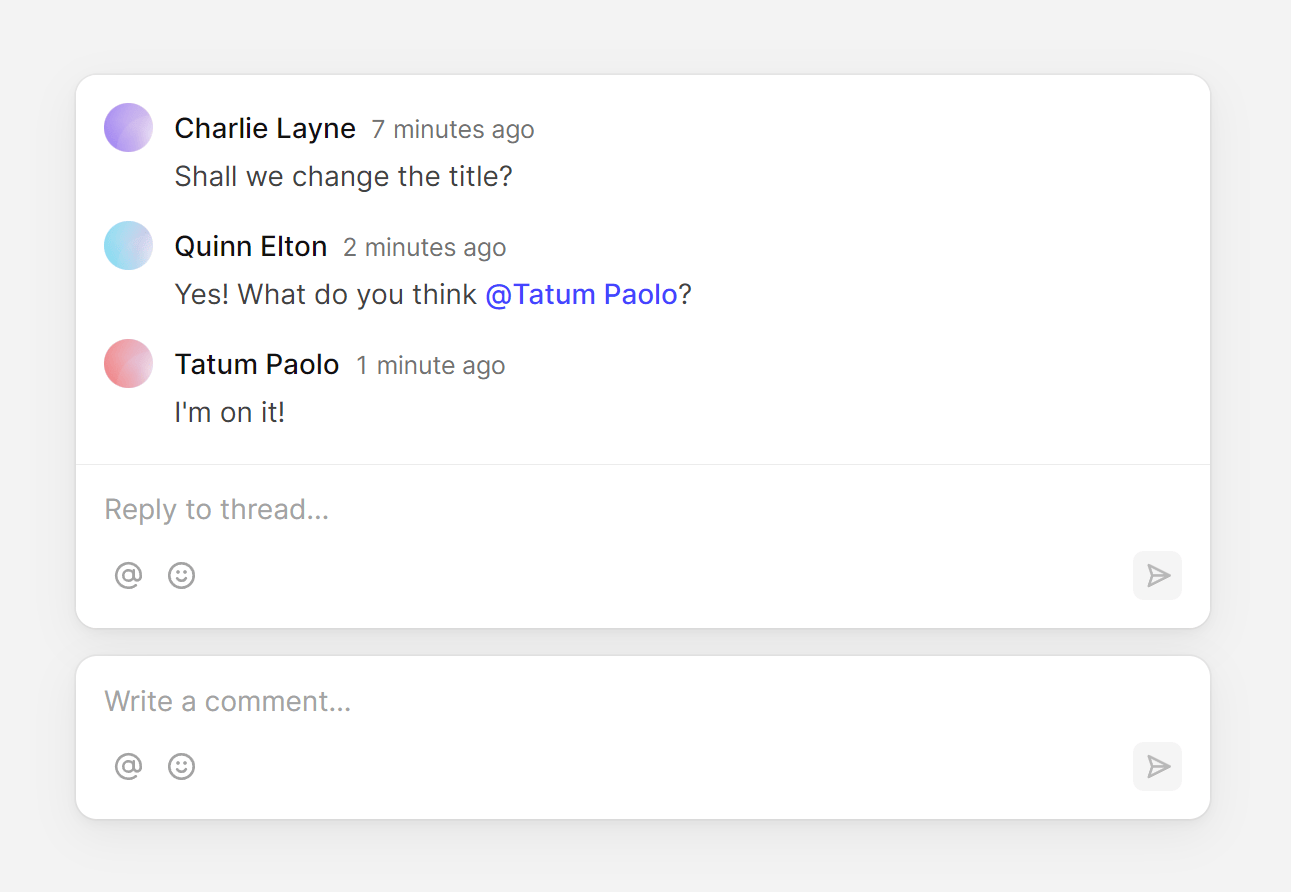
Add the function to your LiveblocksProvider
The
resolveUsers
function is passed as an option toLiveblocksProvider
—let’s add it. This function provides you withuserIds
, an array of user IDs that have interacted with Comments. TheseuserIds
match the IDs set when authenticating users in your app.Return your users
resolveUsers
requires you to return a list of users in theUserMeta["info"]
format we set earlier. Remember that name and avatar are required for the default components, but you can also use any other metadata in your app.We’re only returning one user here, but make sure to return an array containing each user, in the same order you received the IDs.
Real-world example
In your real application you’ll probably be getting users from your API endpoint and database via
fetch
. This is how we’d recommend building out this function.Users are now visible
After adding this, you should now be able to see your users in threads!
Resolving mention suggestions
We can see the users that have commented, but we don’t have a way to search for
users to mention, for example after typing the @
character. We can create a
simple search that resolves this data with
resolveMentionSuggestions
.
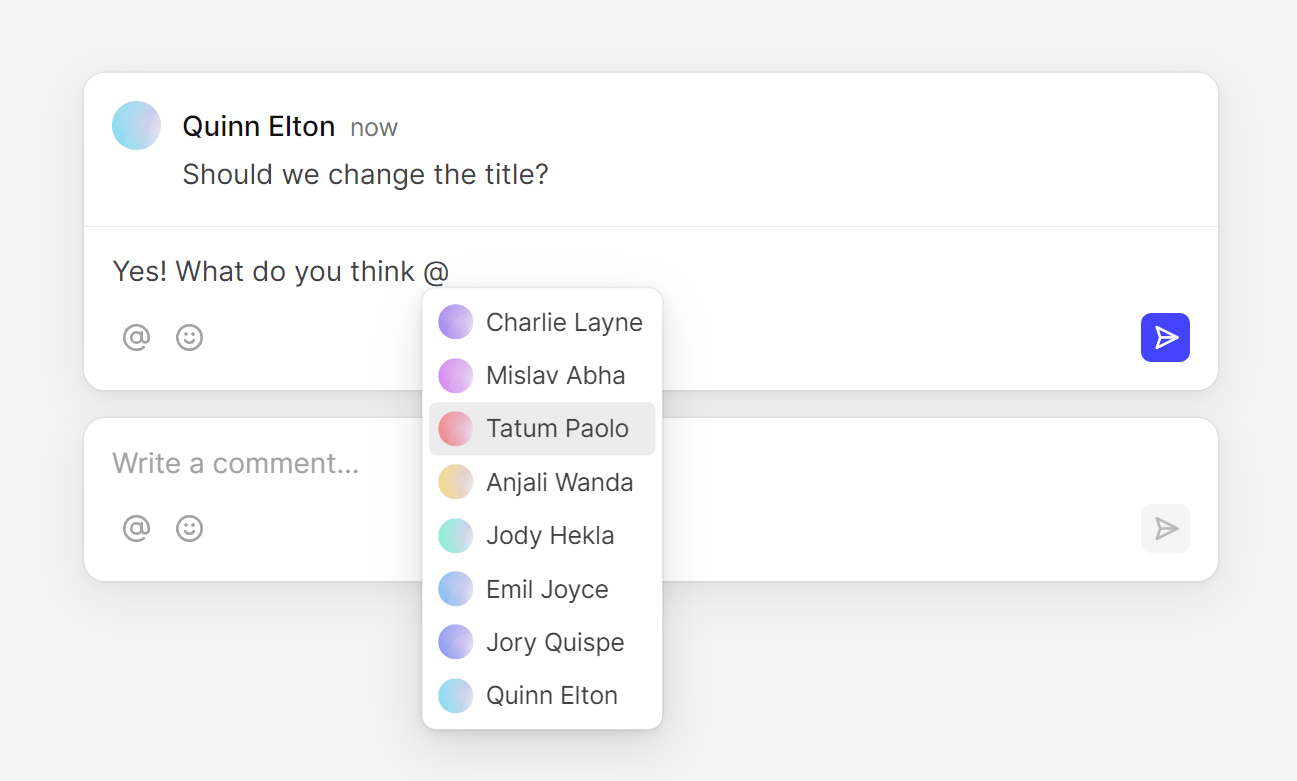
Add the function to your config file
resolveMentionSuggestions
is placed alongsideresolveUsers
, and provides you withtext
, which is the string that the user is searching for. You can use this string to return a list of matching user IDs.Real-world example
In a real application, you’ll most likely be getting a list of each user, before filtering the list by the user’s names or IDs. If
text
is an empty string, then you need to return a list of every user, instead of a filtered list.Mention suggestions now appear
Now we’ve found and returned the correct users, Comments can display a list of mention suggestions!
Next steps
You’re now ready to start building your Comments application! Here’s where you can learn more: