Build share dialogs, filterable overview pages, and a whole lot more with REST APIs
We've added new API endpoints that enable you to build core parts of multiplayer products such as share dialogs with permissions, filterable overview pages, groups with permissions, and more.
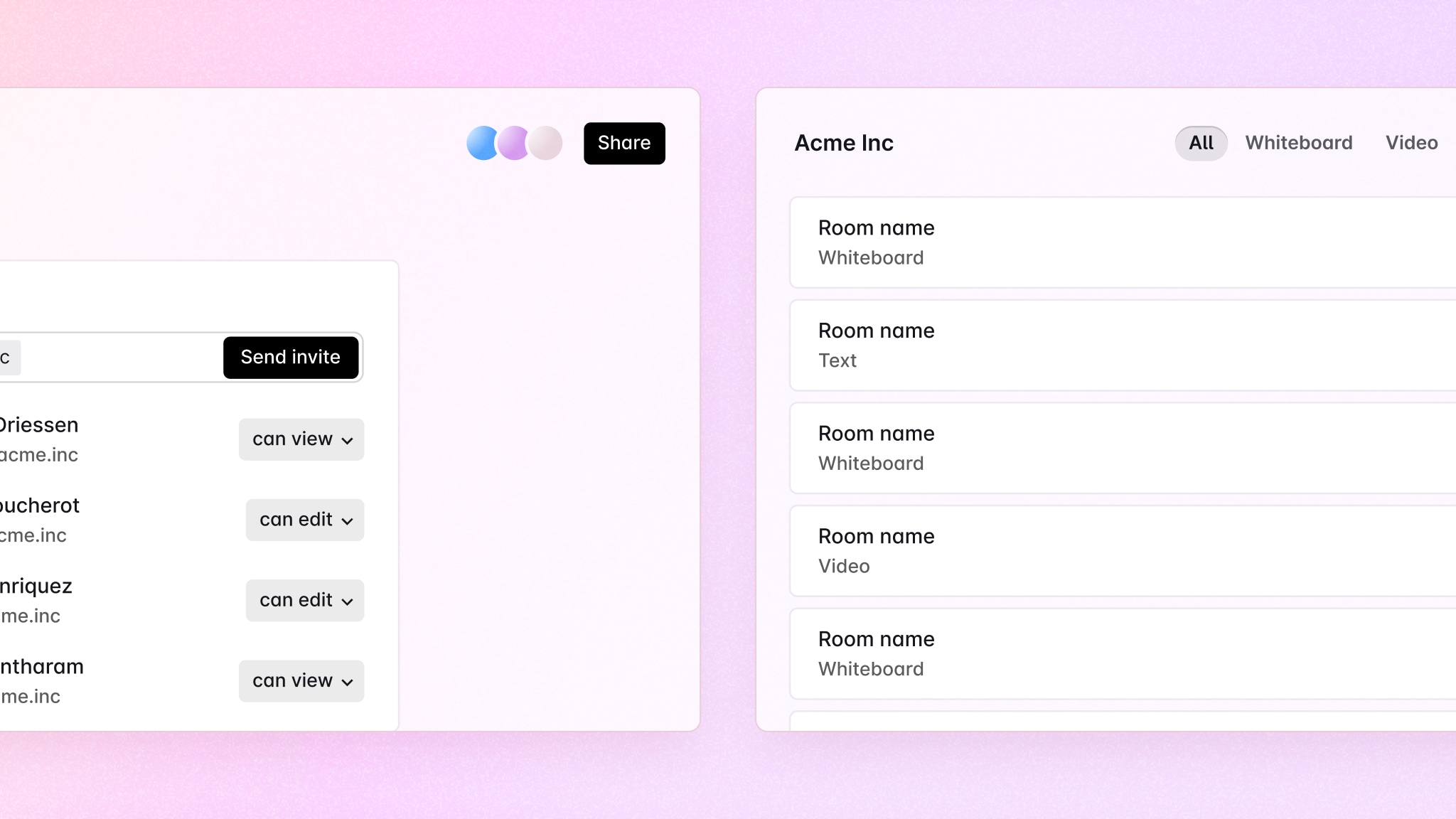
At Liveblocks, our mission is to empower companies and developers to create amazing document‑based collaborative products to enable people to feel more connected and work better together.
The first foundational step to accomplish this mission was about distributing tools and APIs that made it dead simple for developers to build multiplayer editor experiences. What do we mean by that? Think of the Figma’s, Notion’s, and Pitch’s of the world.
But outside of their collaborative editors, what do all those products also have in common? They have a great document/file browsing experience, a smooth share/invite flow driving organic product‑led growth, custom group/user permissions, and more!
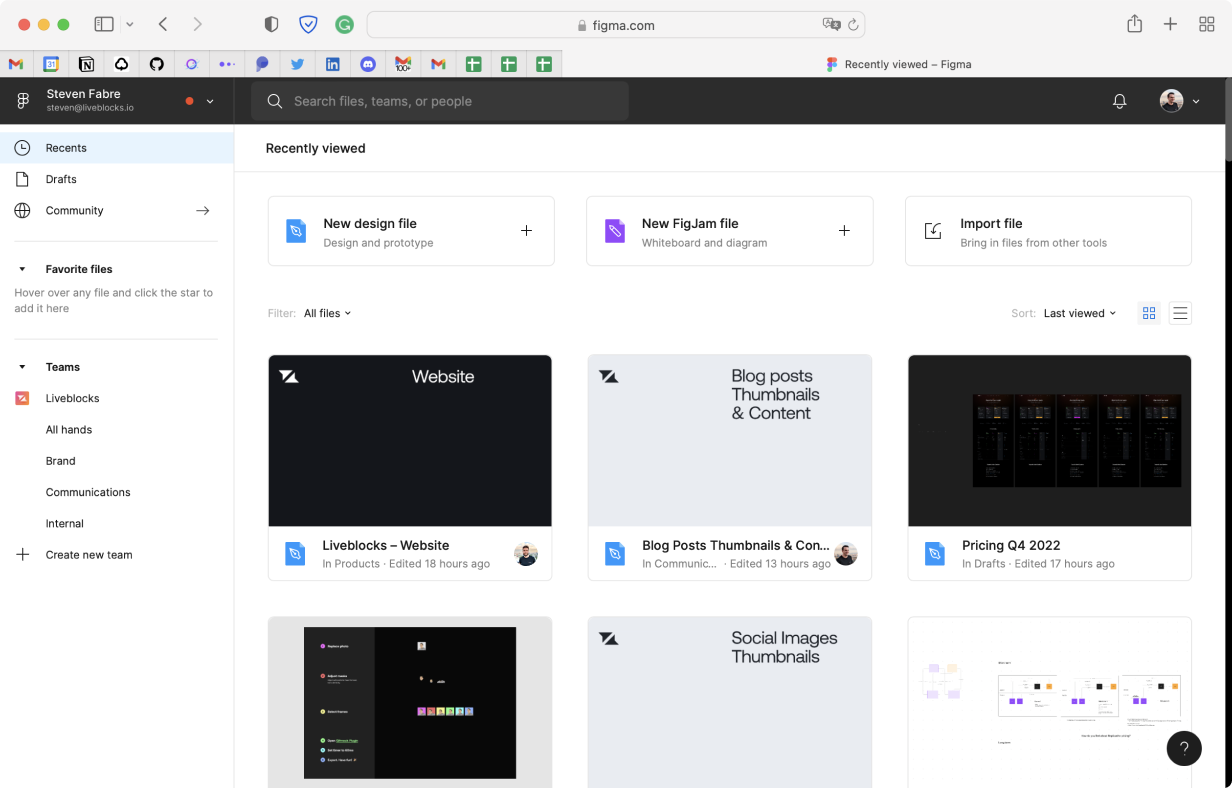
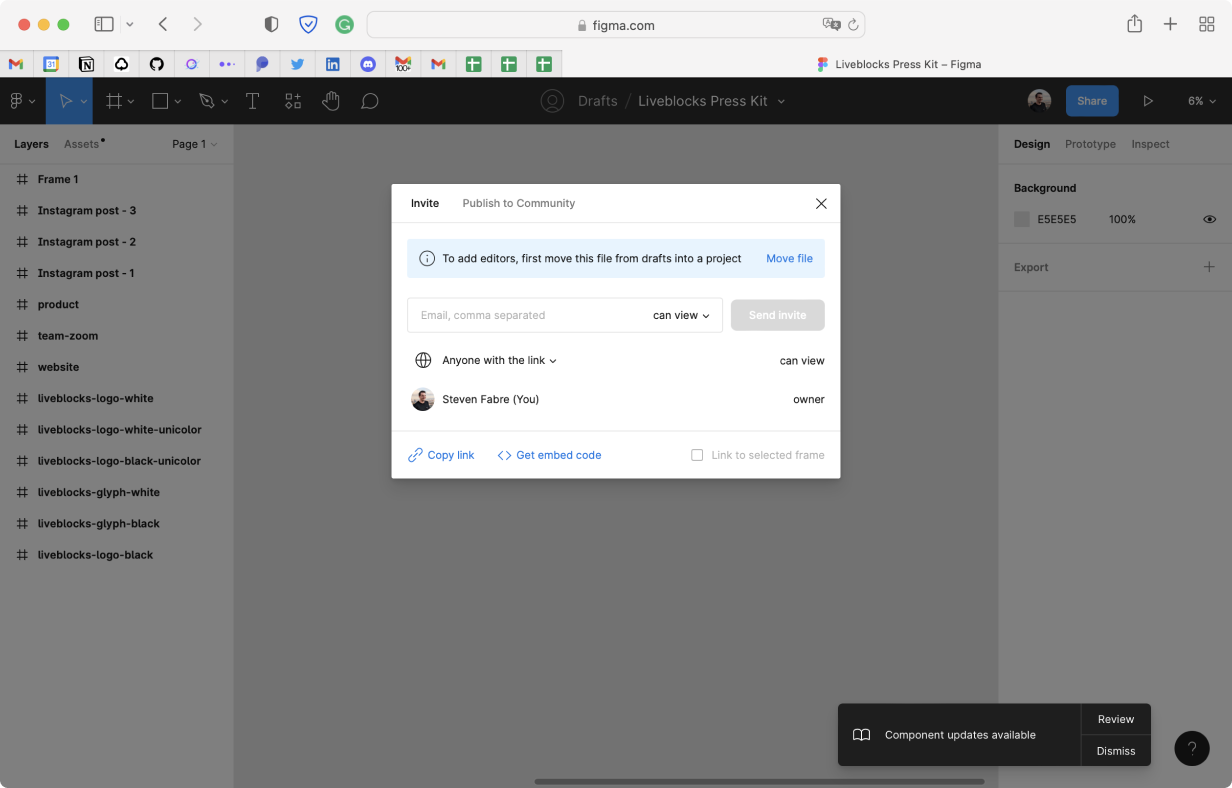
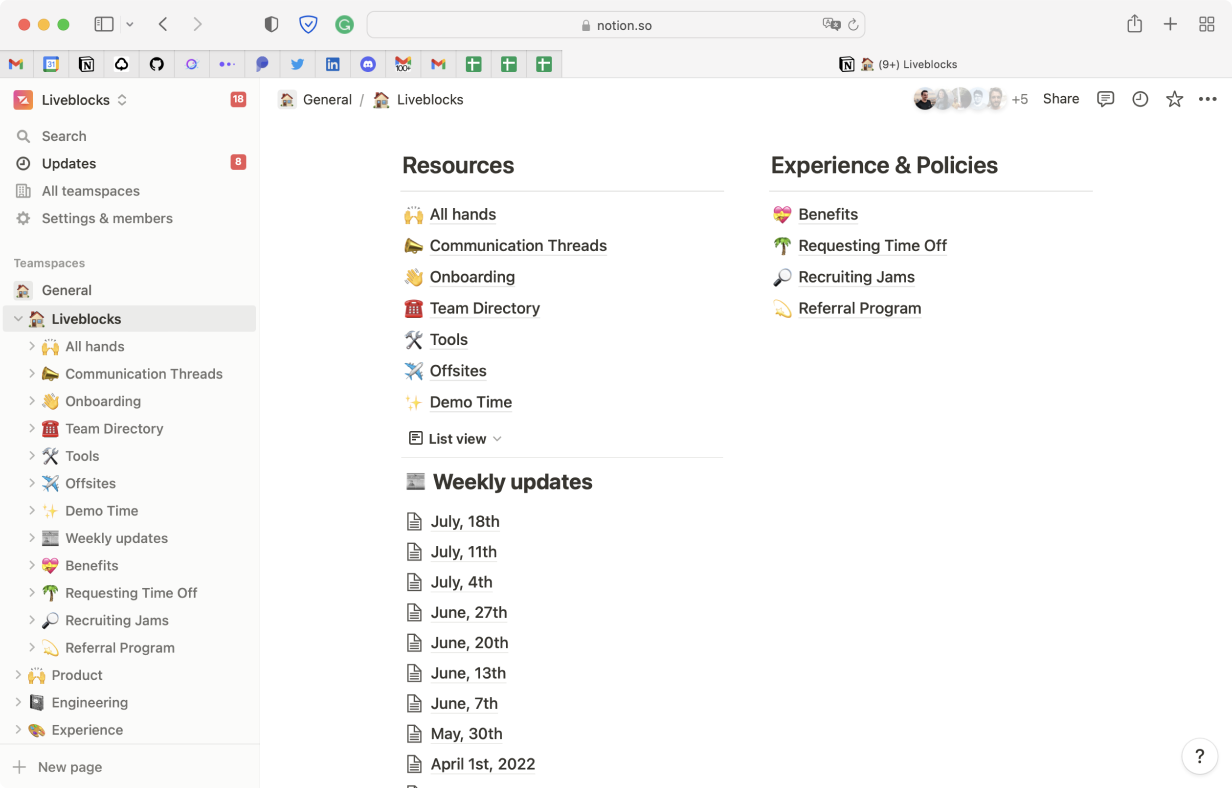
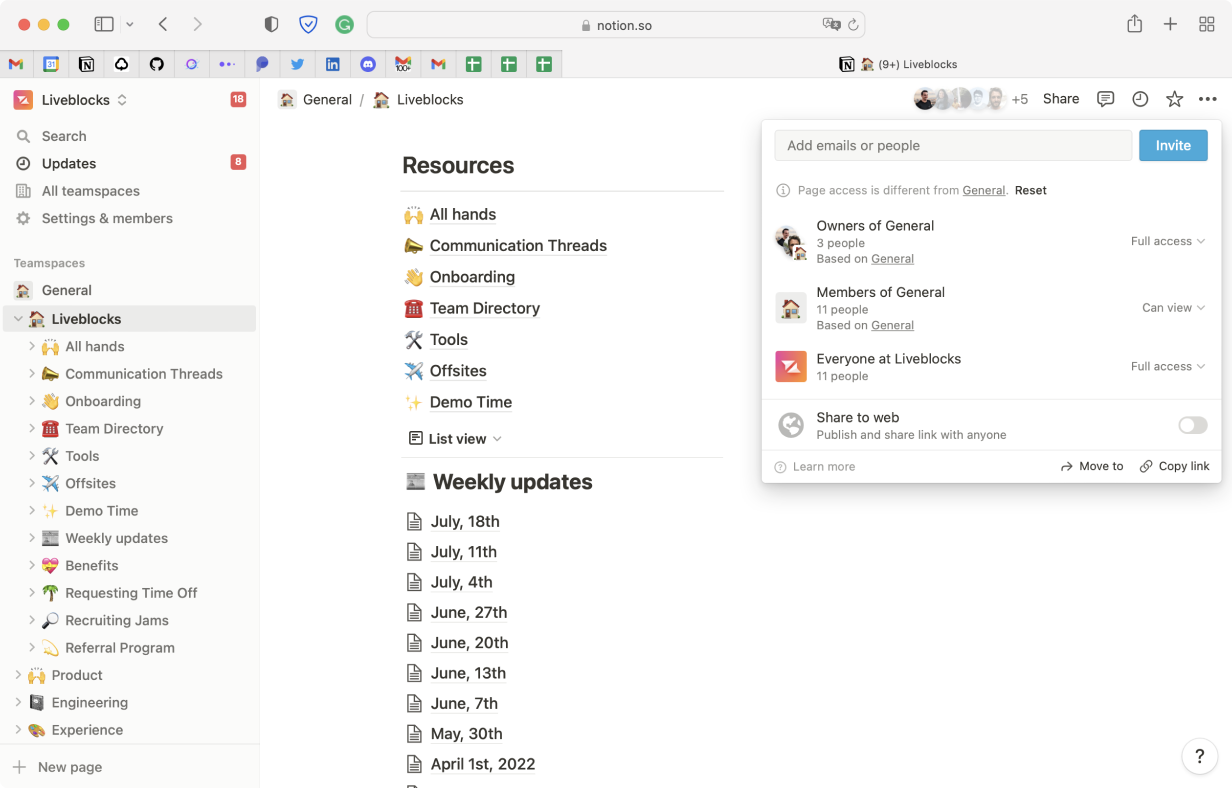
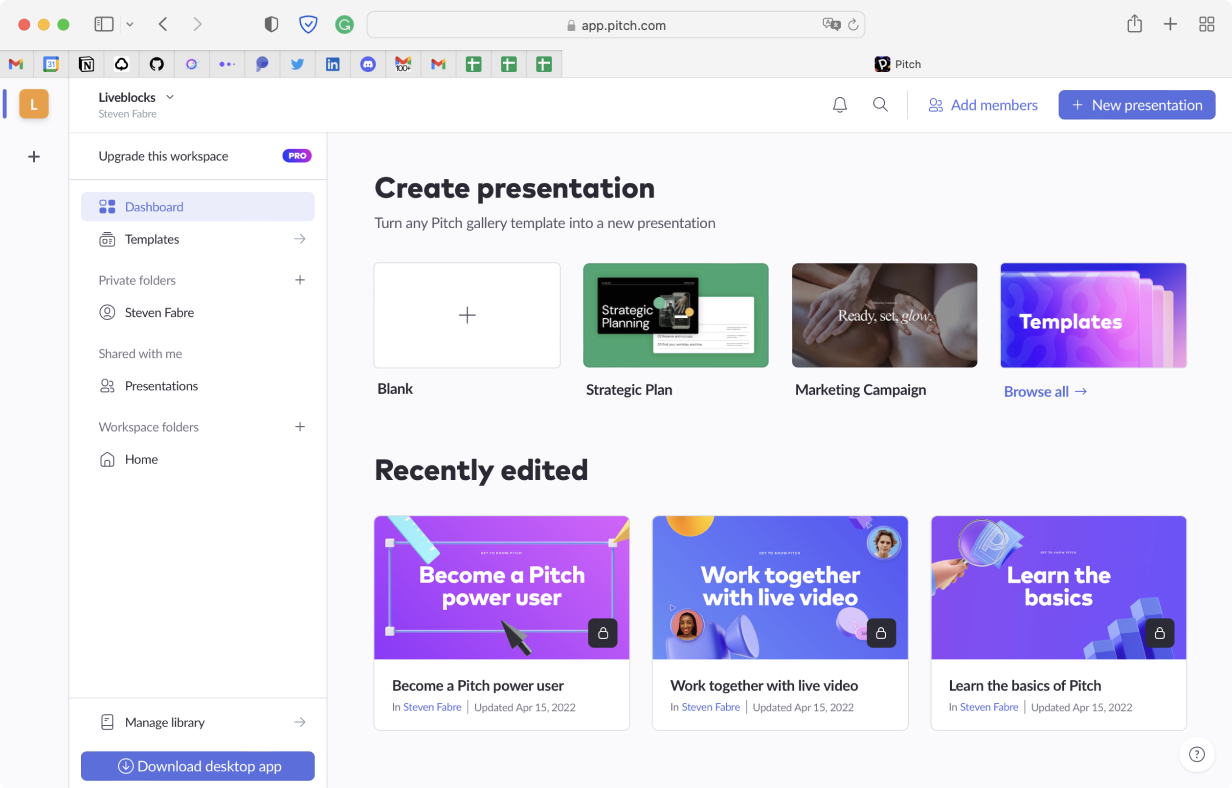
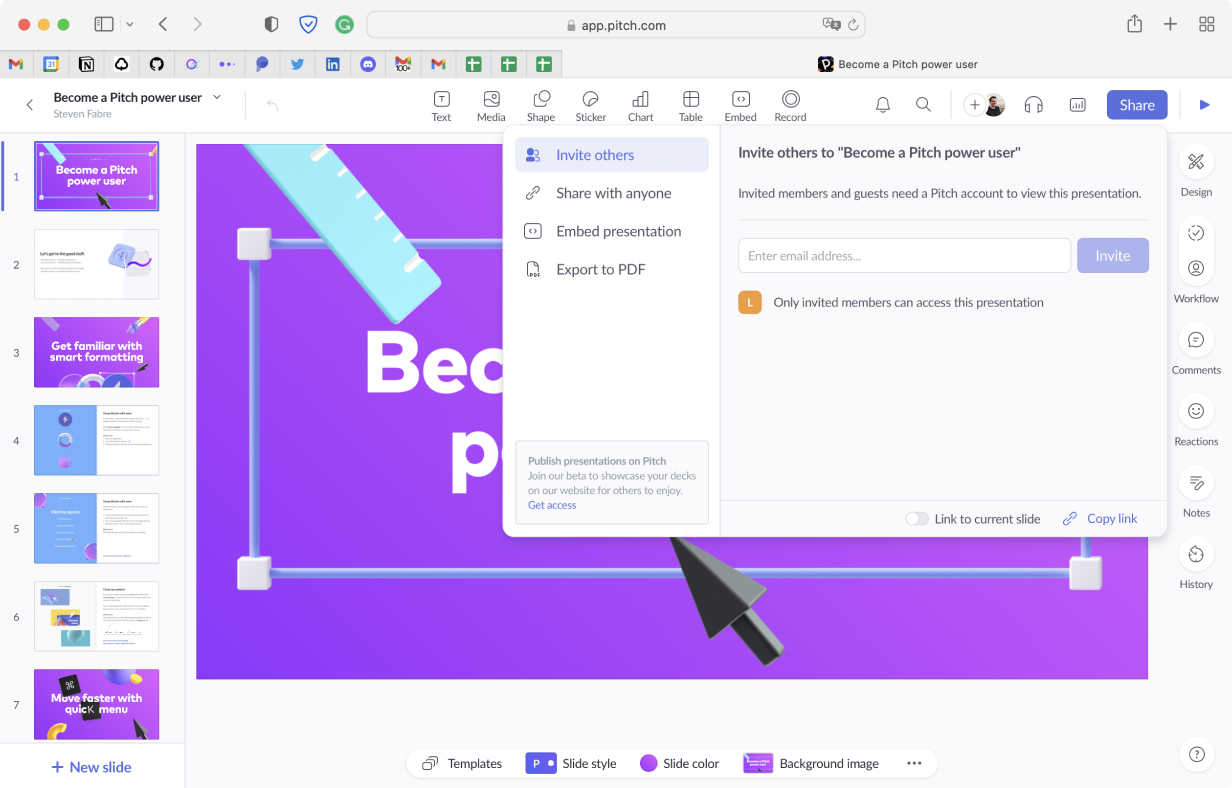
Today, we’re excited to announce a new set of REST APIs that will help you build those critical experiences too. The possibilities are endless, but here are a few common collaborative UI patterns we’d like to highlight today:
The first major improvement is that rooms (or documents in this context) can have different permission types assigned at three different levels: default, groups, and users. We designed this solution to be easy-to-use, yet powerful and flexible, enabling companies to design and build permission systems that fit their unique requirements.
For instance, you can now easily add a world-class share dialog that will organically drive more people to your product.
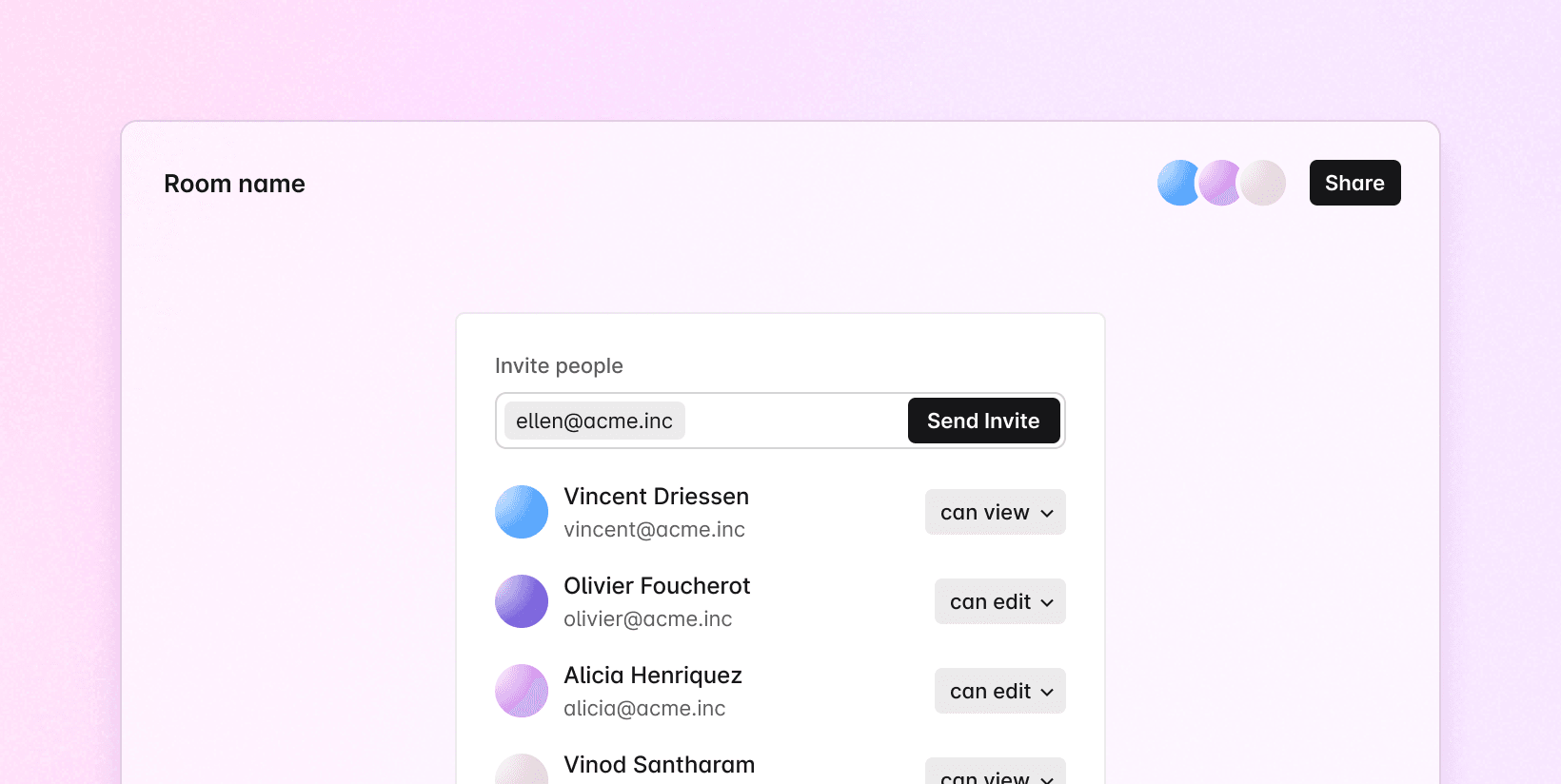
To create the invitation system above, first we need to give a user a userId
when authenticating, for example within
authorize
from @liveblocks/node
.
Then we can use the update room API to assign permissions to that user.
To check a user’s assigned permission types for this room, we can then use the
get room API and
check usersAccesses
.
The invitation system can also be extended to groups of people too, for instance you could set permissions for the "engineering" group. To do this, first give users their groups when authenticating.
Then, give the "engineering" group "room:read"
and "room:presence:write"
access to the document.
The engineering group now has access to view the room, and to edit their presence (which can be used to display an online avatar).
To check if a user only has read-only access to storage in your app, the
isReadOnly
boolean can be also retrieved from others
or self
.
Filterable and paginated overview pages
With this update, rooms can also have custom metadata associated with them, enabling you to build filterable and paginated overview pages.
For instance, if you have different document types in your product, it is now easy to expose those types as a filter—making it easy for people to find what they’re looking for.
One way to edit metadata is to use the
create room API. In this
example, we’re creating a new room with a custom type
metadata, and setting it
to "whiteboard".
We can then use get rooms API to retrieve a list of rooms containing the "whiteboard" type.
And if you have hundreds of documents created, Liveblocks automatically takes
care of pagination by returning the nextPage
URL in the response—making it
easy to fetch the next page by either clicking on a button or some sort of
infinite scrolling behavior.
Groups with permissions
With our new API endpoints, you can also create fully dedicated views for groups. Groups are a common pattern in seat-based collaborative products and are often used to organise documents for different teams and projects.
For instance, if you want to enable your users to configure groups, you could easily list them in side panel in your product overview page.
We’d then use the get rooms API to retrieve a list of rooms the group has access to.
Contributors
This month, we want to send a special shout-out to Vinod Santharam and Florent Lefebvre for their contribution. If you want to learn more about all the API endpoints we released, please check the API Reference. See you soon for the next update!
Ready to get started?
Join thousands of companies using Liveblocks ready‑made collaborative features to drive growth in their products.