Initialize your Liveblocks configuration file with a single command
You can now use a single command to initialize your Liveblocks configuration file, enabling you to start building collaborative experiences quicker than ever.
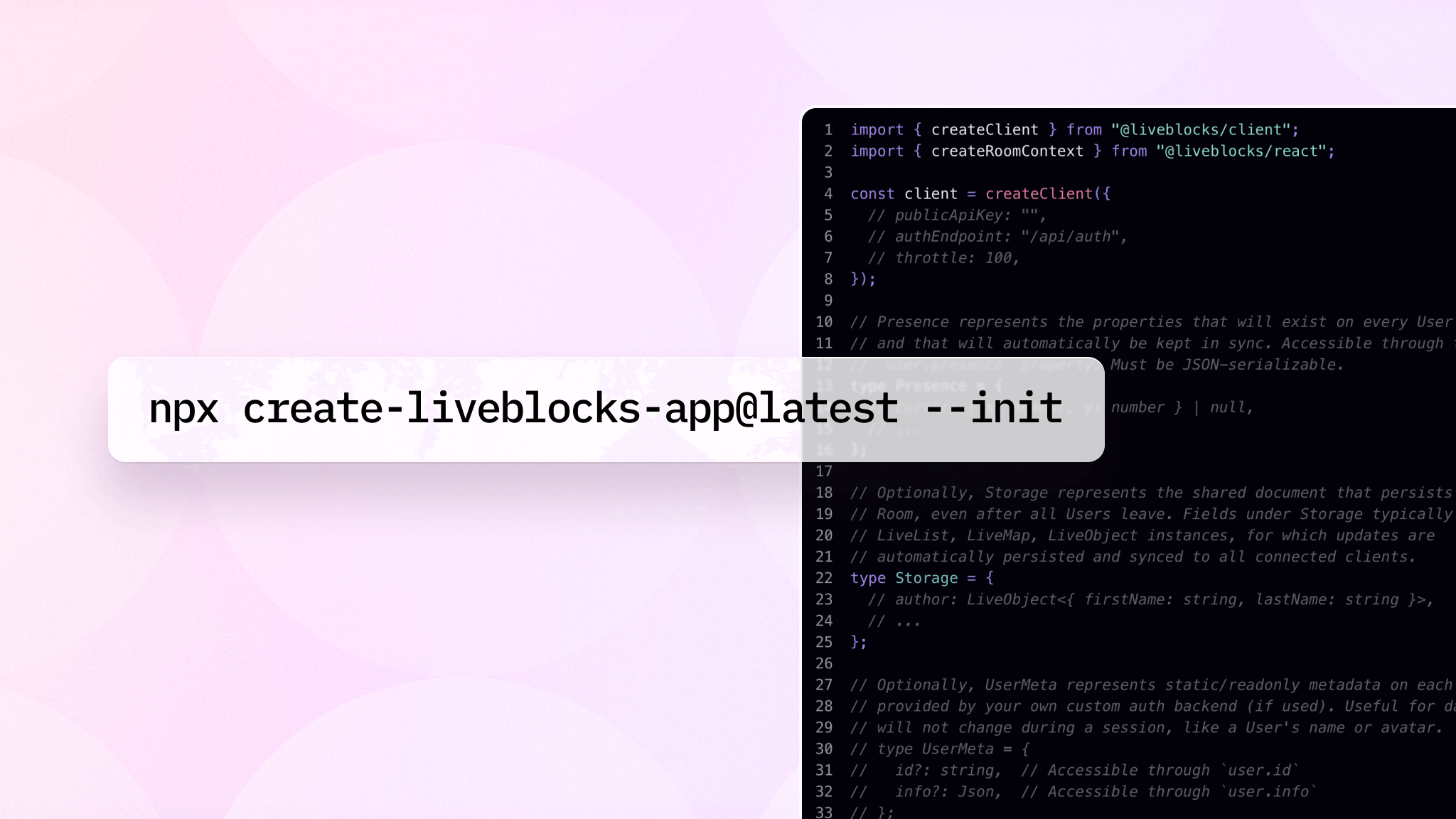
Today, we’re launching a new feature that enables developers to initialize their Liveblocks config files with a single command.
Configuration files
Liveblocks allows you to place all your TypeScript types in a single config
file, liveblocks.config.ts
, and have them propagate throughout your
application. This is made possible by passing your types to the
createRoomContext
function for React.
After adding this, you can then export all your hooks from
liveblocks.config.ts
, and have automatic typing inside your app.
Generating the file
Previously, engineers had to write liveblocks.config.ts
files manually before
integrating Liveblocks into the core of their applications. This made onboarding
slower than it needed to be, especially for people that wanted to try Liveblocks
for the first time.
It now only takes a few seconds to generate a full liveblocks.config.ts
file
by running the following command in your application folder.
Here’s what running that command looks like for a React Suspense configuration.
This will generate the following liveblocks.config.ts
file automatically for
you, enabling you to quickly start building multiplayer experiences for your
product.
You can then start using the Liveblocks RoomProvider
and hooks to build your
realtime app, such as a collaborative editor. For instance, here’s the React
code for listing the connectionId
of everyone currently connected to the same
room.
Updating your config for live cursors
One of the simplest use cases for Liveblocks is live cursors, and how is how you
can integrate it into your React application. In most scenarios, you’d need to
adjust the Storage
, Presence
, RoomEvent
, and UserMeta
types to fit your
needs, but live cursors only needs Presence
, which could look like this.
You can then update people’s cursor positions with updateMyPresence
from the
useMyPresence
hook. To display other people’s cursors, simply loop through the
response from useOthers
and display a custom React component that renders SVG
cursors with the appropriate X and Y coordinates.
That’s all you need—you now have live cursors in your React application!
If you’d like to make your product multiplayer like Figma, Notion, and others, feel free to create an account, read our docs or skim through our GitHub repository. We can’t wait to see what you make with Liveblocks!
Ready to get started?
Join thousands of companies using Liveblocks ready‑made collaborative features to drive growth in their products.