How to create a collaborative to-do list with React and Liveblocks
In this 15-minute tutorial, we’ll be building a collaborative to-do list using React and Liveblocks. As users edit the list, changes will be automatically synced and persisted, allowing for a list that updates in realtime across clients. Users will also be able to see who else is currently online, and when another user is typing.
This guide assumes that you’re already familiar with React, Next.js and TypeScript. If you’re using a state-management library such as Redux or Zustand, we recommend reading one of our dedicated to-do list tutorials:
The source code for this guide is available on GitHub.
Install Liveblocks into your project
Install Liveblocks packages
Create a new app with create-next-app
:
Then run the following command to install the Liveblocks packages:
Join a Liveblocks room
Liveblocks uses the concept of rooms, separate virtual spaces where people
collaborate. To create a realtime experience, multiple users must be connected
to the same room. Create a file in the current directory within /app
, and name
it Room.tsx
. Pass the location of your endpoint to LiveblocksProvider
.
Show who’s currently in the room
Now that Liveblocks is set up, we can start using the hooks to display how many users are currently online.
We’ll be doing this by adding useOthers
, a selector hook that provides us
information about which other users are online.
useOthers
takes a selector function that receives an array, others
,
containing information about each user. We can get the current user count from
the length of that array. Add the following code to pages/index.tsx
, and open
your app in multiple windows to see it in action.
For a tidier UI, replace the content of styles/globals.css
file with the
following css.
Show if someone is typing
Next, we’ll add some code to show a message when another user is typing.
Any online user could start typing, and we need to keep track of this, so it’s
best if each user holds their own isTyping
property.
Luckily, Liveblocks uses the concept of presence to handle these temporary states. A user’s presence can be used to represent the position of a cursor on screen, the selected shape in a design tool, or in this case, if they’re currently typing or not.
Let’s define a new type Presence
with the property isTyping
in
liveblocks.config.ts
to ensure all our presence hooks are typed properly.
We can then call useUpdateMyPresence
whenever we wish to update the user’s
current presence, in this case whether they’re typing or not.
Now that we’re keeping track of everyone’s state, we can create a new component
called SomeoneIsTyping
, and use this to display a message whilst anyone else
is typing. To check if anyone is typing, we’re iterating through others
and
returning true if isTyping
is true for any user.
We also need to make sure that we pass an initialPresence
for isTyping
to
RoomProvider
.
Sync and persist to-dos
To-do list items will be stored even after all users disconnect, so we won’t be using presence to store these values. For this, we need something new.
We’re going to use a LiveList
to store the list of todos inside the room’s
storage, a type of storage that Liveblocks provides. A LiveList
is similar to
a JavaScript array, but its items are synced in realtime across different
clients. Even if multiple users insert, delete, or move items simultaneously,
the LiveList
will still be consistent for all users in the room.
First, let's declare a new type Storage
in liveblocks.config.ts
, like we did
for Presence
. This will ensure that our storage hooks are properly typed.
Go back to Page
to initialize the storage with the initialStorage
prop on
the RoomProvider
.
Accessing storage
We’re going to use the useStorage
hook to get the list of todos previously
created.
useStorage
allows us to select part of the storage from the root
level. We
can find our todos
LiveList
at root.todos
, and we can map through our list
to display each item.
Setting storage
To modify the list, we can use the useMutation
hook. This is a hook that
works similarly to useCallback
, with a dependency array, allowing you to
create a reusable storage mutation.
useMutation
gives you access to the storage root, a LiveObject
. From
here we can use LiveObject.get
to retrieve the todos
list, then use
LiveList.push
and LiveList.delete
to modify our todo list. These
functions are then passed into the appropriate events.
Voilà! We have a working collaborative to-do list, with persistent data storage.
Summary
In this tutorial, we’ve learnt about the concept of rooms, presence, and others. We’ve also learnt how to put all these into practice, and how to persist state using storage too.
You can see some stats about the room you created in your dashboard.
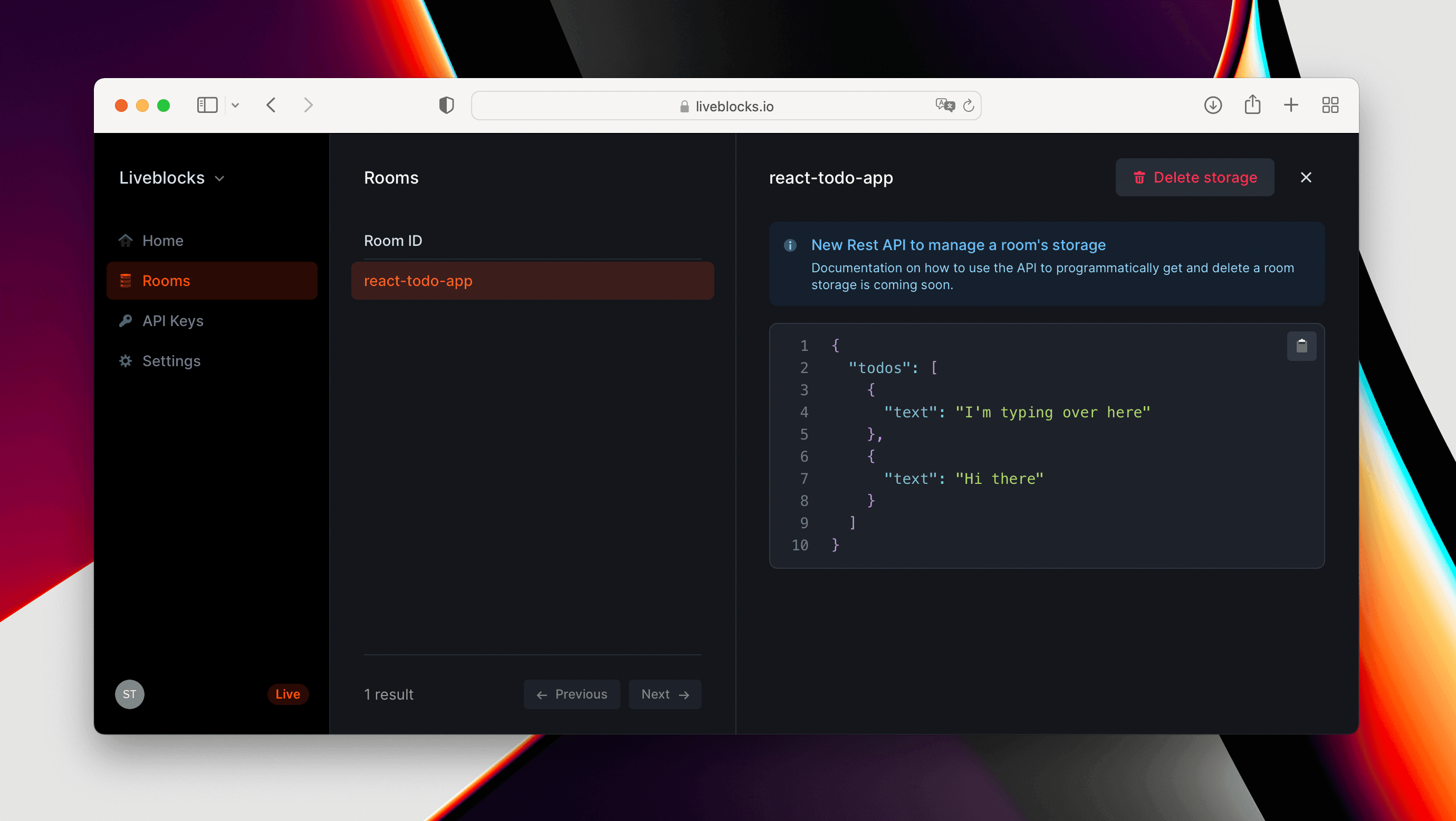