How to send email notifications of unread comments
Liveblocks Comments allows you to build a
commenting experience. With our webhooks and REST API, it’s possible to
aggregate a list of unread comments from the last 30 minutes into a single
email, and send it to your users. Notifications can also be displayed in your
app using
useInboxNotifications
and the
InboxNotification
component.
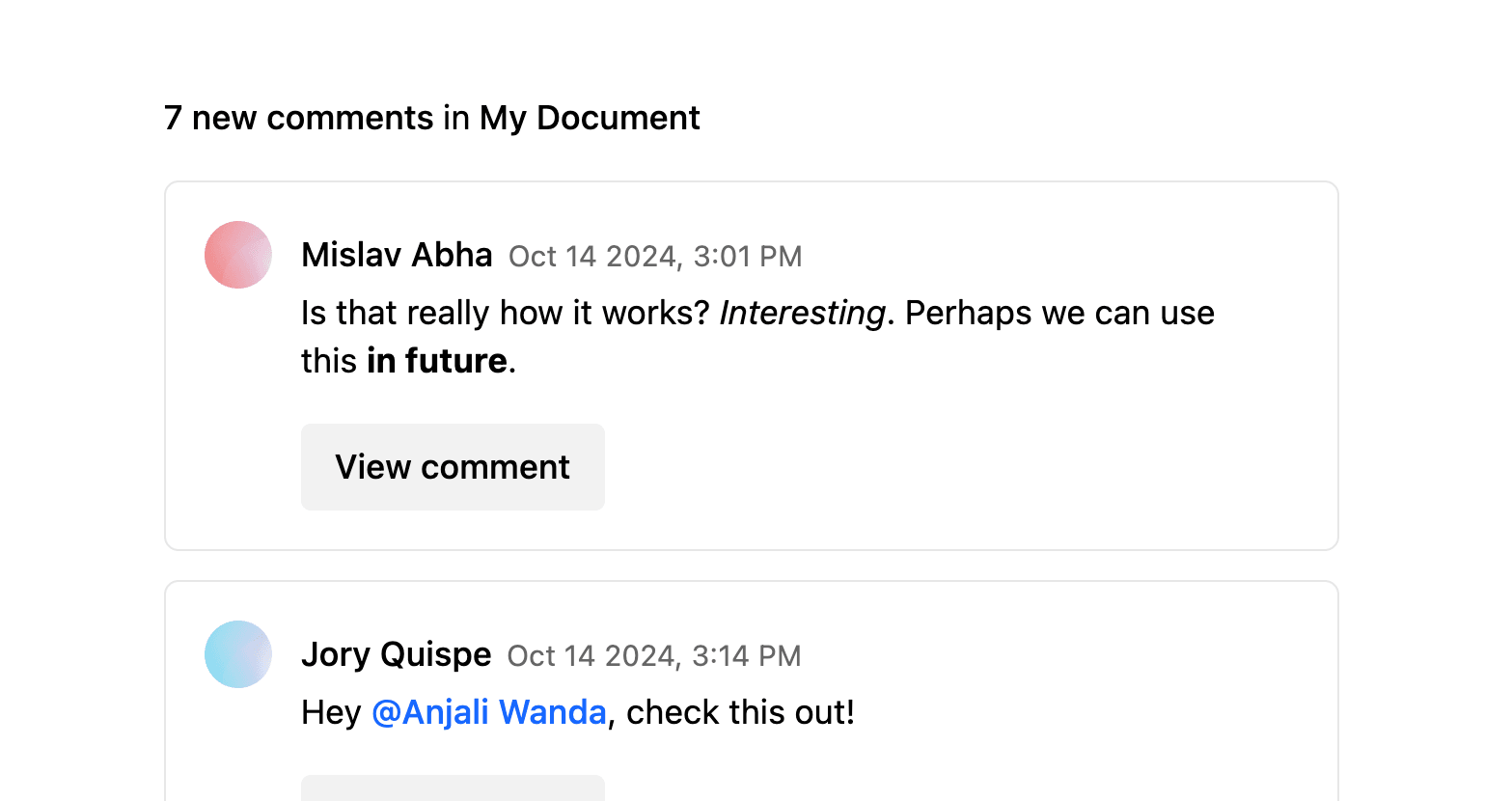
What we’re building
In this guide we’ll be learning how to send emails notifying users about unread comments, and more specifically, we’ll be looking at how to:
- Trigger events based on unread comments using the
NotificationEvent
webhook event. - Fetch unread comments and add styles to comment text using the
@liveblocks/emails
package. - Send an email notification containing a list of unread comments in thread format with Resend.
What are inbox notifications?
Email notifications are built around the concept of inbox notifications, which are different from “normal” notifications in the sense that they can group multiple activities together and evolve over time, which makes more sense when sending email notifications because it helps to avoid sending too many emails. In the case of Comments, inbox notifications are grouped per thread, which means that if there are 4 new comments in a thread you’re participating in, you will have a single inbox notification for it, instead of 4 “normal” notifications.
Learn more about Notifications for Comments in the overview page.
Using webhooks
Liveblocks provides a number of webhooks that can
send requests to your API endpoint when certain events occurs. One webhook we
provide is the NotificationEvent
webhook, which is triggered for each participating user in a thread, 30 minutes
after activity has occurred, and this can be used to send emails to your users.
The information it returns allows you to retrieve comments that have not yet been read by the user, making it possible to aggregate multiple unread comments into a single notification email. Let’s take a look at how to set this up.
Notification channels
You can send notifications via different channels, such as email, Slack,
Microsoft Teams, and Web Push. In our dashboard, you can enable notifications on
certain channels, and in this case, we’ll be using the email channel. You must
always enable the correct channel to ensure your
NotificationEvent
webhook events
are triggered, and this guide will take you through setting it up.
Create an endpoint in your project
When a webhook event is triggered, it can send a POST request to the back end in your project. In this guide, we’ll be using a Next.js route handler (API endpoint) as an example, but other frameworks work similarly.
In order to use webhooks, we’ll need to retrieve the headers
and body
from
the request. Here’s the basic endpoint we’ll be starting from:
Create this endpoint in your project, and make it available on localhost
at
the following URL:
Make a note of this endpoint URL, as you’ll be using it later.
Testing webhooks locally
Running webhooks locally can be difficult, but one way to do this is to use a
tool such as localtunnel
or
ngrok
which allow you to temporarily
put your localhost server online.
If your project is running on localhost:3000
, you can run the following
command to generate a temporary URL that’s available while your localhost server
is running:
localtunnel
generates a base URL that can be placed into the Liveblocks
webhooks dashboard for quick testing. To use this, take the full address of your
webhook endpoint, and replace the domain in your localhost
address with the
generated URL.
You now have a URL that can be used in the webhooks dashboard.
Set up webhooks on the Liveblocks dashboard
To use webhooks, you need to pass your endpoint URL to the webhooks dashboard inside your Liveblocks project, and tell the webhook to trigger when a comment has been created.
Select your project
From the Liveblocks dashboard, navigate to the project you’d like to use with webhooks, or create a new project.
Go to the notifications dashboard
Click on the “Notifications” tab on the menu at the left.
Enable the thread notification type
Click on “Edit” at the top right, enable
thread
notifications on the email channel, and publish your changes.Go to the webhooks dashboard
Click on the “Webhooks” tab on the menu at the left.
Create an endpoint
Click the “Create endpoint…” button on the webhooks dashboard to start setting up your webhook.
Add your endpoint URL
Enter the URL of the endpoint. In a production app this will be the real endpoint, but for now enter your
localtunnel
URL from earlier.Get your webhook secret key
Click “Create endpoint” at the bottom, then find your “Webhook secret key” on the next page, and copy it.
Webhooks dashboard is set up!
Note that you can filter specifically for
notification
events, but we’re ignoring this for now so we can test more easily. Let’s go back to the code.
Verify the webhook request
The @liveblocks/node
package provides
you with a function that verifies whether the current request is a real webhook
request from Liveblocks. You can set this up by setting up a
WebhookHandler
and
running verifyRequest
.
Make sure to add your “Webhook secret key” from the Liveblocks dashboard—in a real project we’d recommend using an environment variable for this.
Check the event and notification permissions
After verifying the request, we can then check we’re receiving the correct type
of event, on the correct channel. There are different notification
events, and
in this case we’d like to check for
thread notification, as we’re
specifically listening for new comments. We can do this using
ThreadNotificationEvent
,
making sure to check for the email
channel.
Note that we’re also checking if the user should receive a notification, and getting their email address—Liveblocks doesn’t have knowledge of your permissions system on the back end, so it’s your responsibility to check if this user should have access to the room.
Fetching data for emails
@liveblocks/emails
provides functions
for fetching unread comments and styling emails, returning them as either React
components or an HTML string. In this guide we’ll use the React function, but
the HTML function works almost identically, so you can still follow along if
you’d prefer HTML emails.
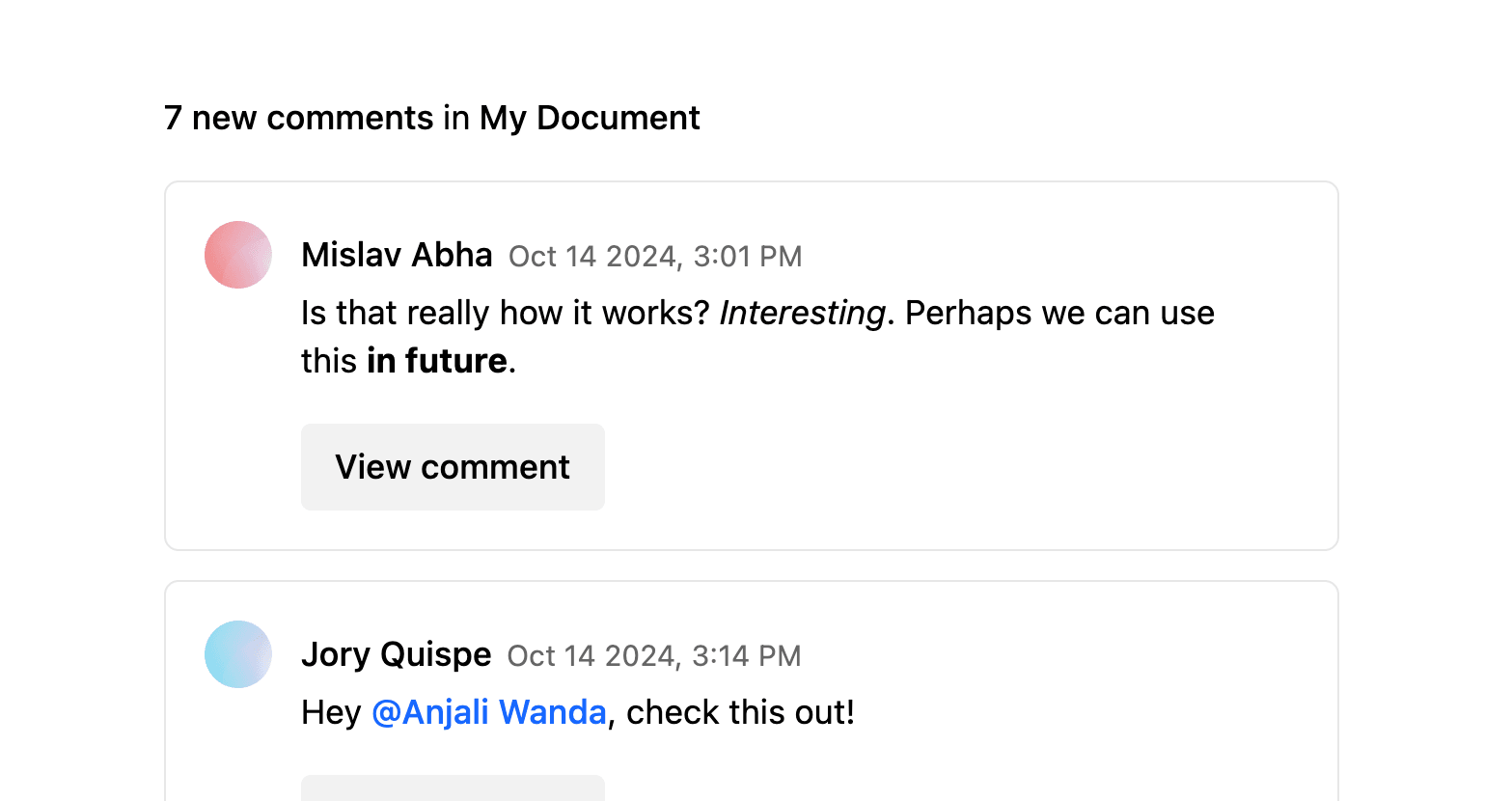
First set up your
Liveblocks
Node.js
client and wrap
prepareThreadNotificationEmailAsReact
in try/catch
, getting the data for the email.
Create the emails
Next, we need to create the emails with React.
prepareThreadNotificationEmailAsReact
helps you identify two different thread notification types, unread replies in
a thread, or an unread mention in a comment. We can choose to create different
emails for these cases.
Resolving data
We’ve now fully created a basic React email, and it’s ready to send. However,
we’re displaying each user’s ID, and not their names. We can go back to
prepareThreadNotificationEmailAsReact
and use resolver functions to transform an ID into a name, for example
steven@example.com
-> Steven
. These functions work similarly to
resolvers on the client.
Customizing comment components
We can also edit prepareThreadNotificationEmailAsReact
to allow for
custom components in comment bodies,
for example we can add margin around a paragraph, color mentions, and underline
links.
Any component can be passed here, including those used in
react-email
,
learn more.
Send notification emails
Now that the React code has been generated, we can send the notification emails. Resend is a great tool for easily sending emails, and in this code example, we’re using it to send notifications to each user. Make sure to add your API key from the Resend dashboard before running the code.
Allow users to toggle notifications
Using Liveblocks hooks and methods, it’s possible to create a notifications settings interface, allowing end users to choose which notifications they’d like to receive, and on which channels, saving their preferences.
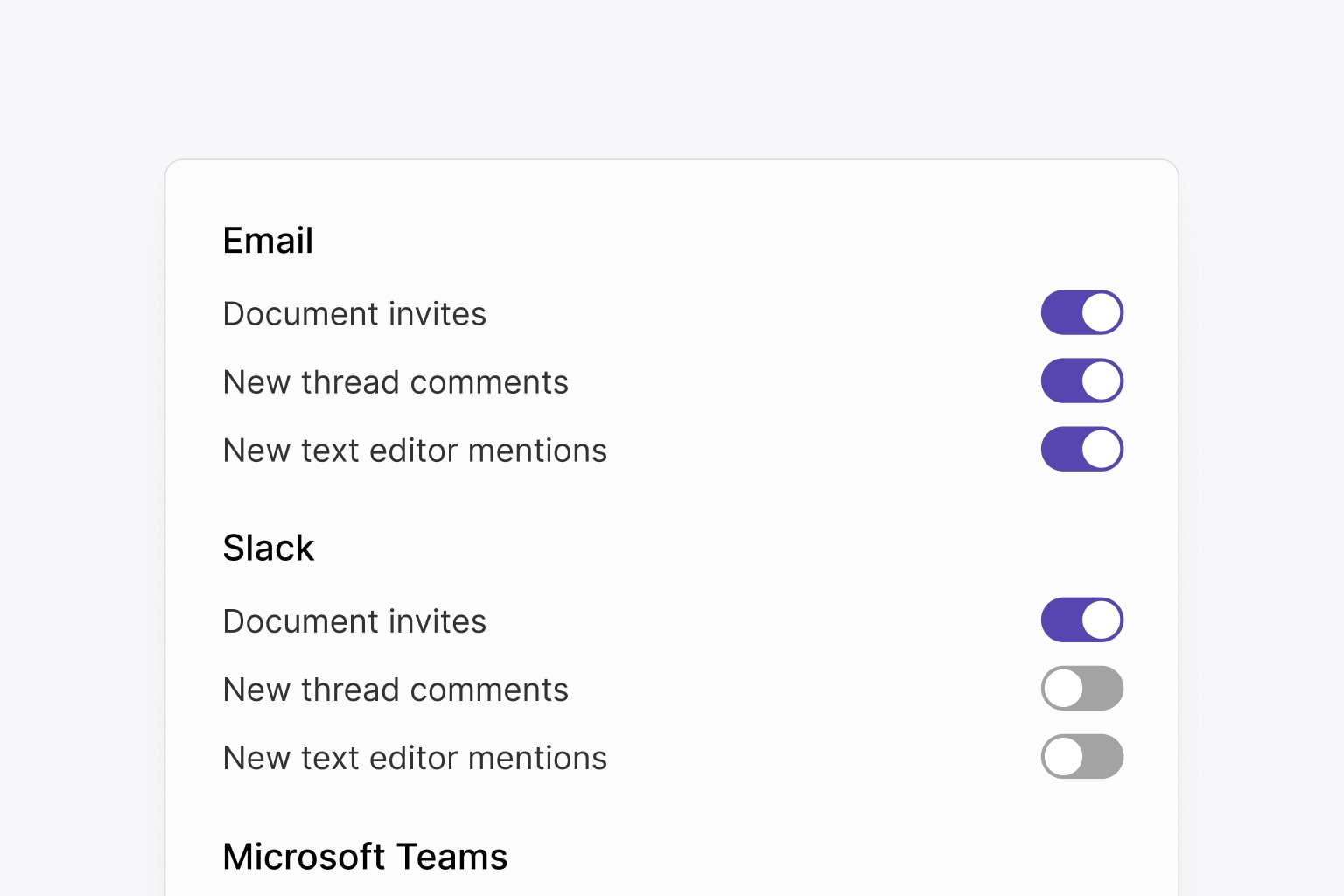
Learn more in our guide on creating a notification settings panel.
Recap
Great, we’re successfully sending email notifications after new comments are created! In this guide we’ve learned:
- How to use webhooks and the
NotificationEvent
. - How to use the
@liveblocks/emails
package to fetch and render unread thread data. - How to send email notifications with Resend.