What to check before enabling a new notification kind
When publishing changes to your notification settings in the dashboard, you should make sure your app is ready to handle any webhooks changes. This is not a problem when disabling a notification kind, but when you enable a new notification kind you should check that your app is ready to receive these new notifications.
Enabling in the dashboard
When in the notifications settings dashboard, you can enable and disable various
webhook events for different kinds. For example, below we’ve toggled a custom
notification kind
.
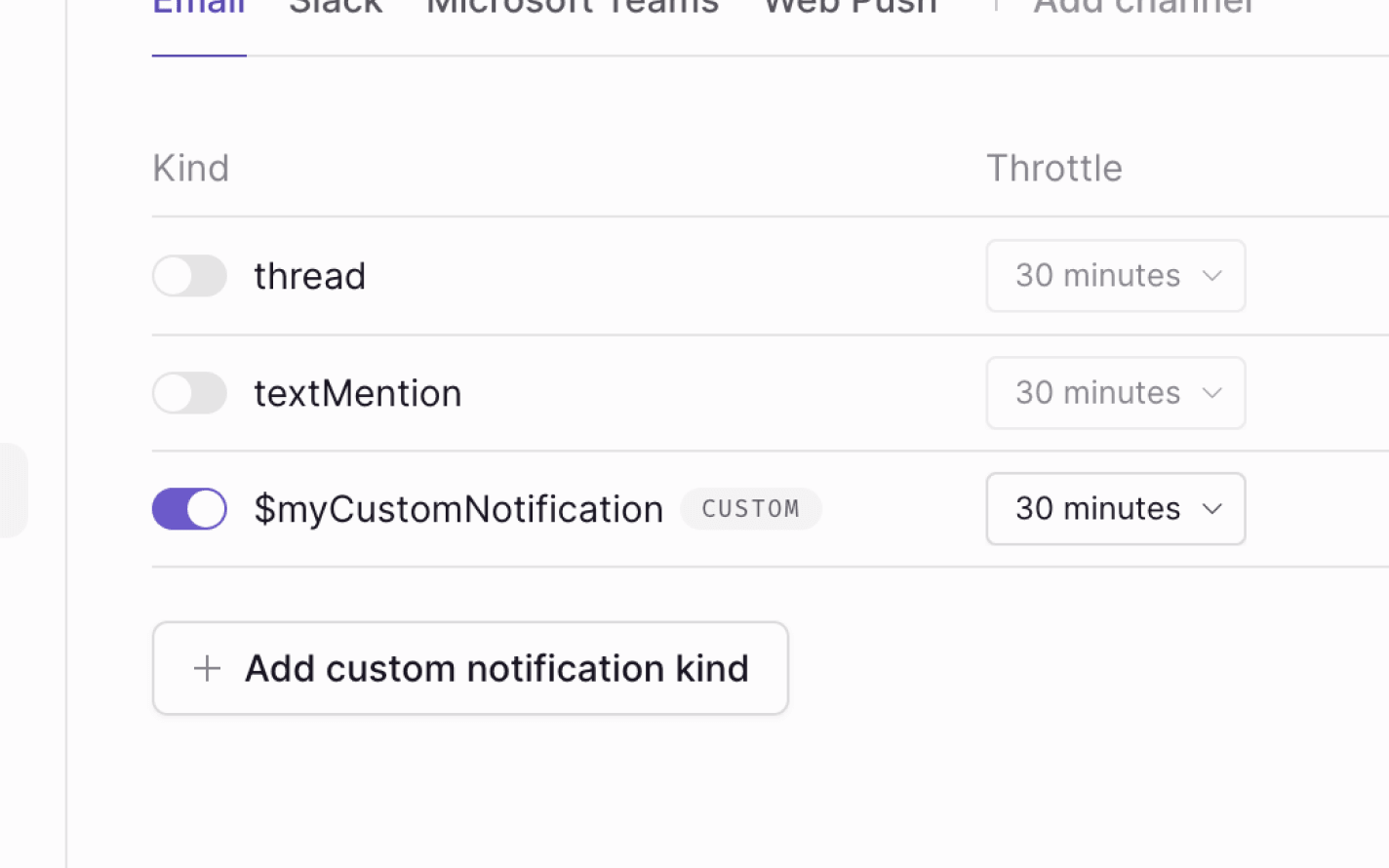
Before publishing this change, it’s important to understand what will occur, and to modify your app.
What happens when you enable a notification kind
After enabling and publishing a notification kind, a new webhook event will be
sent for that kind
on the channel you selected. Below is an example of an API
endpoint set up for a Liveblocks webhook—you can see the new event that will be
received if you were to enable a custom notification sent on the email channel.
As you can see above, the two highlighted lines are the fields for the new event, and you’ll need to handle them.
Before publishing the change
Before publishing your notification settings change, you’ll most likely wish to
check for channel
and kind
in your webhook endpoint, and handle it
accordingly.
If you don’t handle this, you may find yourself running into problems, depending on the way you’ve written the logic in your app.
Safe to publish
After changing your webhook endpoint, it’s safe for you to go back to the notification settings page, and publish your changes.
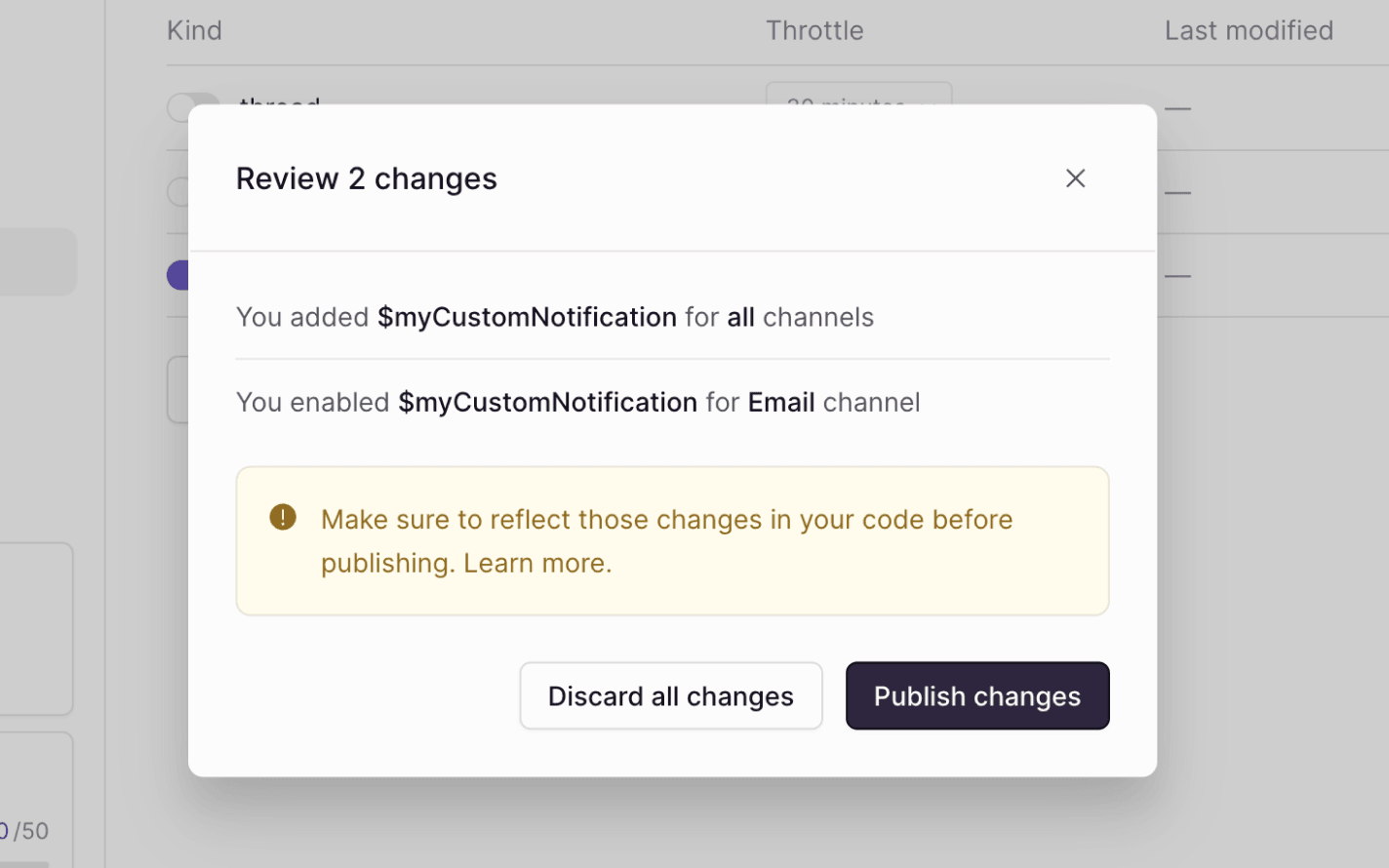
Events for the new notification kind will now be called in your app.
Users can change their preferences
Each user in your app can set their own preferences for notifications, and after enabling a notification kind, each user’s will be set to the default value.
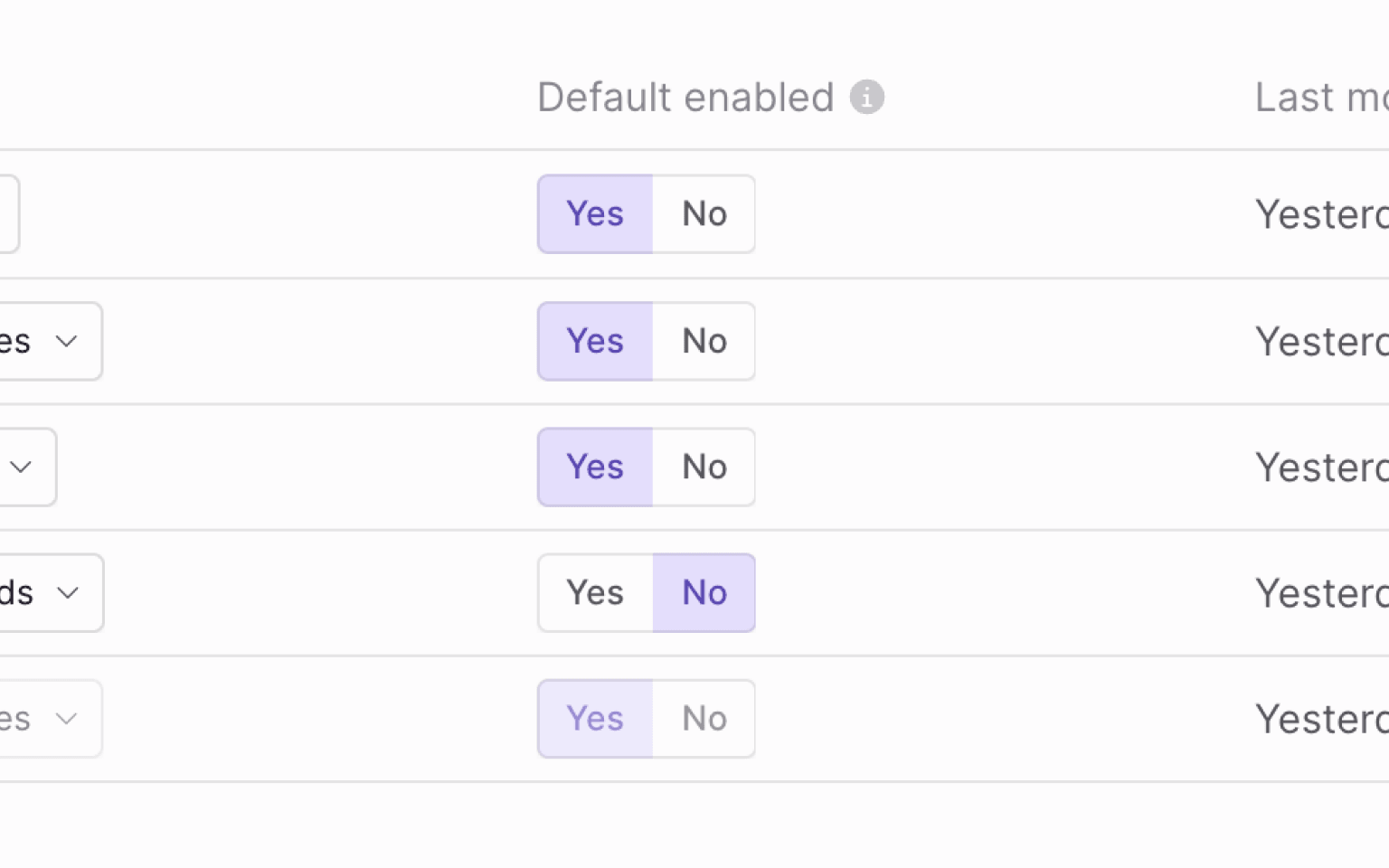
You can use
useUpdateNotificationSettings
to view and set each user’s individual values, making it easy to create
notification setting panels. Below, we’re allowing users to toggle thread
notifications on the email
channel.
You can use this hook to create a full notification panel for each user. If you’re not on React, you can use JavaScript or Node.js functions instead.